

If you’re waiting for data input, for example, a goroutine can fire off to supply pre-populated text even as the user is typing. For web apps, goroutines let you run concurrent jobs while avoiding roadblocks. This feature alone is worth the price of admission. Want to see it in action? Check out this snippet at the Go Playground and you’ll see just how goroutines work. Like the aforementioned vine, think of goroutines as little flowers that branch off and die while the main vine keeps going. The hello() function could be anything-a data call, a transaction, a queue entry-and it would run while the rest of the program churns along. The program finishes when the main routine wakes up and fires off a Println. All that’s important is the work is done. If anything were to happen in the hello() function, the main function wouldn’t notice. The program takes a quick nap and then continues. In this code, main fires off a goroutine-indicated by the go keyword-called hello(). The main function works just like it does in C. Take a look at this simple example: package main Complete database failure? Your goroutine will know, and you can gracefully work around the issue. Network timeout? Don’t worry, your goroutine will manage it while the main loop continues. In other words, you can fire off a goroutine, have it run, and keep going while it and thousands of its brethren process in the background. These are essentially concurrent functions that run while the rest of the program completes. Built for concurrencyīefore we dig in further, let’s look at goroutines. Further, its multithreading capabilities-most specifically, its goroutines-are a surprising and welcome addition to your bag of tricks. Because Go was designed to run on multiple cores, it is built to support concurrency and scale as cores are added. Running Go on modern hardware-and even inside containers or on virtual machines-can be a real pleasure. Their frustration at their toolset forced them to rethink system programming from the ground up, creating a lean, mean, and compiled solution that allows for massive multithreading, concurrency, and performance under pressure. The story goes that Google engineers designed Go while waiting for other programs to compile. With its growing popularity, there are a number of reasons you should take a closer look at it. That said, Go has slowly but surely inundated the development world like a creeping vine, covering everything that came before it in a lush-and in many ways superior-cover of programming power. Notice that we need to use a then callback to make sure the second message is logged with a delay.To paraphrase the indie band Cracker, what the world needs now is another programming language like I need a hole in the head.
#PHP SLEEP ALTERNATIVE CODE#
This code will log “Hello”, wait for two seconds, then log “World!” Under the hood, we’re using the setTimeout method to resolve a promise after a given number of milliseconds.
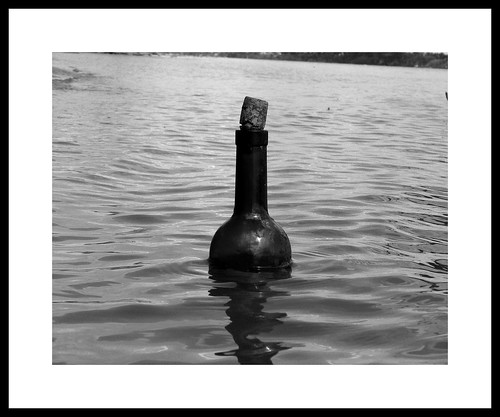
The standard way of creating a delay in JavaScript is to use its setTimeout method. Now that we have a better understanding of JavaScript’s execution model, let’s have a look at how JavaScript handles delays and asynchronous code.
#PHP SLEEP ALTERNATIVE HOW TO#
How to Use SetTimeout in JavaScript Properly If any of this is news to you, you should watch this excellent conference talk: What the heck is the event loop anyway? Rather, it will continue on its way, output “Hello!” to the console, and then when the request returns a couple of hundred milliseconds later, it will output the number of repos. It will not, however, wait for the request to complete. The JavaScript interpreter will encounter the fetch command and dispatch the request. This is because fetching data from an API is an asynchronous operation in JavaScript.
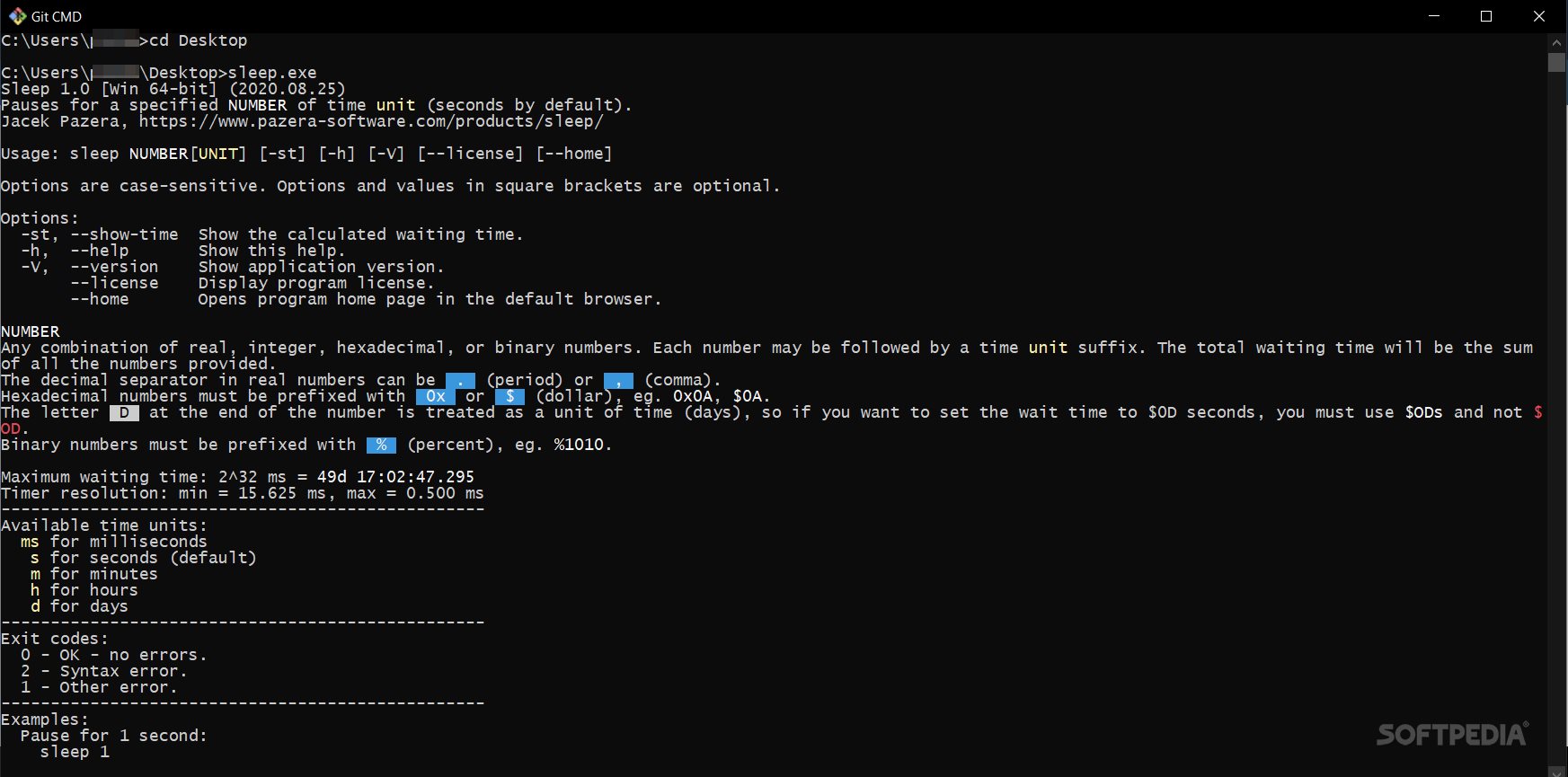
If you run this code, it will output “Hello!” to the screen, then the number of public repos attributed to my GitHub account. Execution goes from top to bottom.Ĭontrast that with the equivalent JavaScript version: fetch ( '' ). It then parses the response, outputs the number of public repos attributed to my GitHub account and finally prints “Hello!” to the screen. get (uri ) )Īs one might expect, this code makes a request to the GitHub API to fetch my user data. Understanding this is crucial for effectively managing time and asynchronous operations in your code.Ĭonsider the following Ruby code: require 'net/http' require 'json' Now that we’ve got a quick solution under our belts, let’s delve into the mechanics of JavaScript’s execution model. Understanding JavaScript’s Execution Model
